Today we're going to solve another Coding Challenge. This one is categorized as: "intermediate" Coding Challenge. Uuuuuh
Personally I don't think that this is that much of an "intermediate" challenge, but... doesn't really matter, the important thing is that we have to solve this too, right?
This is also coming from FreeCodeCamp and it says:
You will be provided with an initial array (the
first
argument in the destroyer function), followed by one or more arguments. Remove all elements from the initial array that are of the same value as these arguments.Note: You have to use the
arguments
object.
So basically we'll have to create a function called destroyer
. When it will be called they'll provide an array
or numbers
as the first argument and then one or more arguments as numbers
. We need to remove all the occurrences of these numbers from the array
.
Example
In order for us to visualize it better let's see an example of what the function must do:
destroyer([1, 2, 3, 2, 3, 1], 2, 3)
- Should return [1, 1]
as we remove all the 2
's and 3
's from the provided array
.
Easy peasy lemon squeezy. Right?
Unlike this little cute kitty which doesn't know how to tackle the situation, we know, right? xD Or if we don't know, we'll learn... together! (I'm talking about solving the coding challenge, not about how we can squeeze a lemon... for that we'll have to wait for the kitty to do it first and then teach us).
The Solution
First thing first, let's write the function:
function destroyer(arr) {}
Now, let's make a plan. As I told you in the previous post, it's importat to break down the steps before writing any code. This will simplify the process.
- Get all the
values
from thearguments
object using Object.values - Remove the first item as it is the provided
array
- Save all the remaining numbers into a separate array, let's call it
destroyerArr
- Filter out all the
numbers
that are into thearr
by comparing them to the numbers from thedestoryerArr
.
It might sound a little confusing with all these arrays, but let's continue and once you'll see the code, it will be much simpler:
function destroyer(arr) {
// Step 3. // Step 1. // Step 2.
const destroyerArr = Object.values(arguments).slice(1);
// Step 4.
return arr.filter(x => !destroyerArr.find(y => y === x));
}
As you can see in Step 4 we used the find
method to check if the current item from the arr
(the x
) is in the destroyerArr
. If it is, the find
function will return it and by adding !
in front of it, we'll convert it to a boolean and negate it.
Might be confusing, but let's see how that'll work:
- when the
find
function will return a number, like:2
for example, by adding!
in front it will be converted tofalse
-> JavaScript "magic". xD - Otherwise if the
find
function will returnundefined
, because it couldn't find the number in thedestroyerArr
, the!
operation will convertundefined
totrue
-> JavaScript "magic" reloaded!
We could also combine all the steps in a nice one-liner. Why doing that? Well.. just because it's fun and I like doing it! xD
function destroyer(arr) {
return arr.filter(
x =>
!Object.values(arguments)
.slice(1)
.find(y => y === x)
);
}
Done!
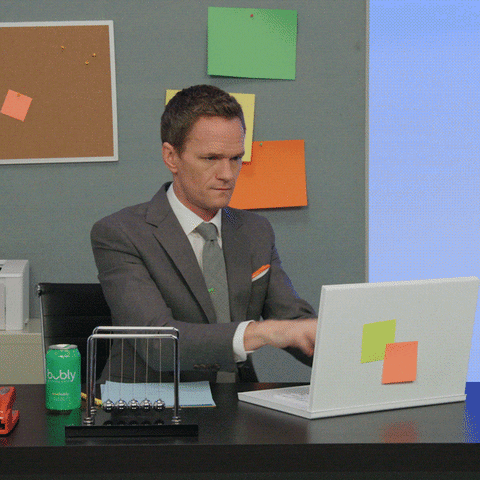
Conclusion
Today's challenge wasn't that hard, but I still enjoyed finding a solution to it.
Can you think of another way to solve it? Maybe a better approach? Let's see what you can find!